Scripts in Paint Shop Pro use a scripting language called Python. In order to perform advanced tasks in PSP scripts, it is necessary to understand Python. This tutorial will cover the if clause (also known as the if statement). Sometimes it is beneficial in a script to allow a user to choose to perform an action or not. This choice occurs using the if clause. I will show how to manipulate a simple script so that a user can choose whether or not to apply a plugin.
Supplies:
Simple Ribbon Tutorial
1. Have my
Simple Ribbon Tutorial available (either printed or open in a web browser).
2. Go to the script toolbar (or menu) and press the circle to start recording.
Toolbar
Menu
3. Follow the directions in the Simple Ribbon Tutorial, including the optional steps. Do not worry if you make a mistake. If you make a mistake, just undo the action (Ctrl-Z).
4. Once you have finished the tutorial, save the script by going to the script toolbar (or menu) and pressing the disk icon.
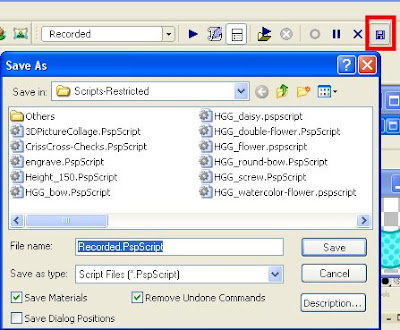
A window will pop up asking you for a name (and location) to save the script. Make sure 'Remove Undone Commands' is checked and 'Save Dialog Positions' is unchecked. To make sure that your script will be identical to the one in this tutorial, make sure 'Save Materials' is checked. You now have a script that will make a ribbon in only one set of colors. If you save the script with 'Save Materials' unchecked, then the script will make a ribbon in your existing foreground and background colors.
5. Now we will edit the script so that the user can choose whether or not to apply the plugin, Greg's Factory Output Vol. II: Pool Shadow. Open up the script editor by clicking the scroll button on the script toolbar.
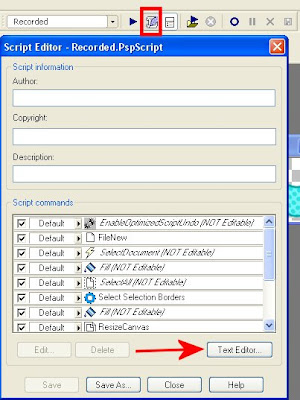
A pop up window for the Script Editor will appear. Go to the bottom and click 'Text Editor.'
6. The script looks like this:
from JascApp import *
def ScriptProperties():
return {
'Author': u'',
'Copyright': u'',
'Description': u'',
'Host': u'Paint Shop Pro 9',
'Host Version': u'9.01'
}
def Do(Environment):
# EnableOptimizedScriptUndo
App.Do( Environment, 'EnableOptimizedScriptUndo', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# FileNew
App.Do( Environment, 'NewFile', {
'Width': 3600,
'Height': 150,
'ColorDepth': App.Constants.Colordepth.SixteenMillionColor,
'DimensionUnits': App.Constants.DimensionType.Pixels,
'ResolutionUnits': App.Constants.ResolutionUnits.PixelsPerIn,
'Resolution': 300,
'FillMaterial': {
'Color': (255,255,255),
'Pattern': None,
'Gradient': None,
'Texture': None,
'Art': None
},
'Transparent': True,
'LayerType': App.Constants.NewLayerType.Raster,
'ArtMediaTexture': {
'Category': u'Art Media',
'Name': u'Asphalt',
'EnableFill': True,
'FillColor': (255,255,255)
},
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# SelectDocument
App.Do( Environment, 'SelectDocument', {
'SelectedImage': 0,
'Strict': False,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Fill
App.Do( Environment, 'Fill', {
'BlendMode': App.Constants.BlendMode.Normal,
'MatchMode': App.Constants.MatchMode.None,
'Material': {
'Color': (54,146,171),
'Pattern': None,
'Gradient': None,
'Texture': None,
'Art': None
},
'UseForeground': True,
'Opacity': 100,
'Point': (2376.5,66.5),
'SampleMerged': False,
'Tolerance': 10,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# SelectAll
App.Do( Environment, 'SelectAll', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Select Selection Borders
App.Do( Environment, 'SelectSelectionBorders', {
'Antialias': True,
'BordersType': App.Constants.BordersType.Inside,
'BorderWidth': 10,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Fill
App.Do( Environment, 'Fill', {
'BlendMode': App.Constants.BlendMode.Normal,
'MatchMode': App.Constants.MatchMode.None,
'Material': {
'Color': (141,204,213),
'Pattern': None,
'Gradient': None,
'Texture': None,
'Art': None
},
'UseForeground': True,
'Opacity': 100,
'Point': (2079.5,3.5),
'SampleMerged': False,
'Tolerance': 10,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# ResizeCanvas
App.Do( Environment, 'ResizeCanvas', {
'AspectRatio': 24,
'FillColor': (255,255,255),
'HoriPlace': App.Constants.HorizontalType.Center,
'MaintainAspect': False,
'NewDimUnits': App.Constants.UnitsOfMeasure.Pixels,
'NewHeight': 150,
'NewWidth': 3580,
'PlaceBottom': 0,
'PlaceLeft': -10,
'PlaceRight': -10,
'PlaceTop': 0,
'VertPlace': App.Constants.VerticalType.Center,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Blinds
App.Do( Environment, 'Blinds', {
'Width': 5,
'Opacity': 25,
'Horizontal': False,
'LightFromLeftTop': True,
'Color': (62,105,148),
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# SelectInvert
App.Do( Environment, 'SelectInvert', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Halftone
App.Do( Environment, 'Halftone', {
'BackgroundColor': (255,255,255),
'ColorScheme': App.Constants.ColorScheme.Greyscale,
'HalftonePattern': App.Constants.HalftonePattern.Line,
'Overlay': True,
'OverlayBlendMode': App.Constants.BlendMode.Screen,
'OverlayOpacity': 48,
'PatternColor': (0,0,0),
'ScreenAngles': (90,137,137),
'Size': 5,
'TransparentBackground': False,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# SelectNone
App.Do( Environment, 'SelectNone', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Magic Wand
App.Do( Environment, 'MagicWand', {
'General': {
'Mode': App.Constants.SelectionOperation.Replace,
'Antialias': True,
'Feather': 0,
'SampleMerged': False
},
'MatchMode': App.Constants.MatchMode.RGBValue,
'Contiguous': False,
'Point': (1482.5,90.5),
'Tolerance': 1,
'AntialiasType': App.Constants.AntialiasType.Outside,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Drop Shadow
App.Do( Environment, 'DropShadow', {
'Blur': 5,
'Color': (0,0,0),
'Horizontal': 0,
'NewLayer': False,
'Opacity': 80,
'Vertical': 0,
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# SelectNone
App.Do( Environment, 'SelectNone', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
}
})
# Gregs Factory Output Vol. II_Pool Shadow
App.Do( Environment, 'Gregs Factory Output Vol. II_Pool Shadow', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
},
'DefaultProperties': []
})
We are going to place the section in purple inside an if clause. First, we need to ask the user a question using a Message Box.
result = App.Do(Environment, 'MsgBox', {
'Buttons': App.Constants.MsgButtons.YesNo,
'Icon': App.Constants.MsgIcons.Question,
'Text': 'Do you wish to apply Greg\'s Factory Output\n'
'Vol. II Pool Shadow?',
})
The \n in the text section tells the Message Box to insert a return. Special characters, such as the apostrophe, need to be escaped with a backslash (\) so that the text does not end prematurely.
7. This will create a question
message box with two options: Yes and No. (
Read more about message boxes.)
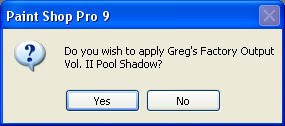
The answer to the question is stored in the variable 'result'. The value of 'result' will either be 1 or 0. If the answer is Yes, then 'result' will be 1. If the answer is No, then 'result' will be 0. By itself, the answer to the question does not cause the script to pause, skip the plugin or perform any action. The answer to the question, 'result' must be used by a conditional (in this case 'if') to produce the desired effect.
8. To make the script use the plugin if the user chooses yes, but skip the plugin if the user chooses no, insert the 'if' statement before the plugin command.
if result == 1:
# Gregs Factory Output Vol. II_Pool Shadow
App.Do( Environment, 'Gregs Factory Output Vol. II_Pool Shadow', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
},
'DefaultProperties': []
})
else:
# MsgBox
Result = App.Do(Environment,'MsgBox',{
'Buttons':App.Constants.MsgButtons.OK,
'Icon':App.Constants.MsgIcons.Info,
'Text':'Script Complete\n\n'
'http://humbuggraphicsgalore.blogspot.com/',
})
This conditional says, if the result is equal to one (meaning Yes) then use the plugin. Else, if the result is not equal to one then produce a message box saying that the script is complete.
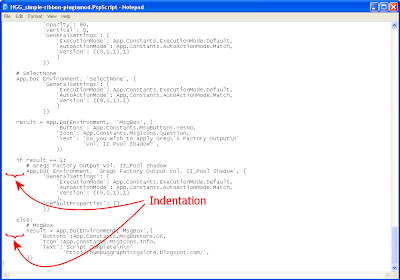
Note that both the plugin command and the message box are nested inside the if clause. Indentation matters.
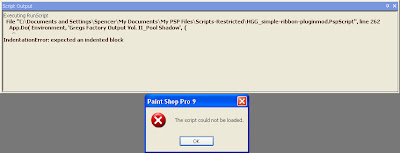
If the block is not indented, then the script will not load and the script output window will produce an error stating, "IndentationError: expected an indented block"
9. The 'else' part of the if clause is optional. It does not need to exist and it only occurs when the user answers 'No' to the question. The following code snippet would produce the same effect for the plugin, but the final message would always appear, not just when the user clicked 'No'.
if result == 1:
# Gregs Factory Output Vol. II_Pool Shadow
App.Do( Environment, 'Gregs Factory Output Vol. II_Pool Shadow', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
},
'DefaultProperties': []
})
# MsgBox
Result = App.Do(Environment,'MsgBox',{
'Buttons':App.Constants.MsgButtons.OK,
'Icon':App.Constants.MsgIcons.Info,
'Text':'Script Complete\n\n'
'http://humbuggraphicsgalore.blogspot.com/',
})
In this case, what happens is that after the script reaches the end of the if clause, it continues with the rest of the script.
10. Finally, multiple if statements can be nested inside each other. For example,
EC5 = App.Do(Environment, 'MsgBox', {
'Buttons': App.Constants.MsgButtons.YesNo,
'Icon': App.Constants.MsgIcons.Question,
'Text': 'Do you have Eye Candy 5 Impact?',
})
if EC5 == 1:
# Alien Skin Eye Candy 5: Impact_Bevel
App.Do( Environment, 'Alien Skin Eye Candy 5: Impact_Bevel', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Default,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((9,0,1),1)
},
'DefaultProperties': [(1313631852,1651470188,0,(1,r'''AQ==''')),(1466201192,1413830740,0,(1,
r'''NDIgcGl4ZWxzAA==''')),(1131312242,1685026146,0,(1,r'''AAAAAACAQUA=''')),(1399682152,
1685026146,0,(1,r'''AAAAAAAAWUA=''')),(1111847523,1685026146,0,(1,r'''AAAAAAAAAAA=''')),
(1111848057,1701737837,0,(1,r'''aXRlQjBUZWI=''')),(1148349294,1685026146,0,(1,r'''AAAAAAAAAAA='''
)),(1181491232,1651470188,0,(1,r'''AQ==''')),(1111843443,1701737837,0,(1,r'''eXR1UzBSdXM='''
)),(1114468417,1685026146,0,(1,r'''AAAAAAAAOUA=''')),(1399874414,1413830740,0,(1,r'''OS4wODMgcGl4ZWxzAA=='''
)),(1382966355,1819242087,0,(1,r'''1x8AAA==''')),(1148347252,1685026146,0,(1,r'''AAAAAADgcEA='''
)),(1229874028,1685026146,0,(1,r'''AAAAAABAUEA=''')),(1114793832,1685026146,0,(1,r'''AAAAAAAAREA='''
)),(1399353968,1685026146,0,(1,r'''AAAAAACAUUA=''')),(1131180576,1413830740,0,(1,r'''UkdCOiAyNTUgMjU1IDI1NSAA'''
)),(1935963971,1413830740,0,(1,r'''UkdCOiA2NCA2NCA2NCAA''')),(1111843683,1413830740,0,(1
,r'''Q3VzdG9tQ3VydmU6IDMgMCAxIDAgMC41IDAuNjYgMCAxIDAgMAA=''')),(1111843700,1701737837,0,
(1,r'''dGNlQjBUY2I=''')),(1111843444,1701737837,0,(1,r'''Y2JlQjBVYmI='''))]
})
else:
EC4000 = App.Do(Environment, 'MsgBox', {
'Buttons': App.Constants.MsgButtons.YesNo,
'Icon': App.Constants.MsgIcons.Question,
'Text': 'Do you have Eye Candy 4000?',
})
if EC4000 == 1:
# Eye Candy 4000_Bevel Boss
App.Do( Environment, 'Eye Candy 4000_Bevel Boss', {
'GeneralSettings': {
'ExecutionMode': App.Constants.ExecutionMode.Interactive,
'AutoActionMode': App.Constants.AutoActionMode.Match,
'Version': ((12,0,1),1)
},
'DefaultProperties': [(1466201192,1413830740,0,(1,r'''MjQuMDAgcGl4ZWxzAA==''')),(1131312242,
1685026146,0,(1,r'''AAAAAAAASUA=''')),(1399682152,1685026146,0,(1,r'''AAAAAADAUkA=''')),
(1651928147,1701737837,0,(1,r'''aXRlQjBUZWI=''')),(1148349294,1685026146,0,(1,r'''AAAAAAAAAAA='''
)),(1181491232,1651470188,0,(1,r'''AQ==''')),(1148347252,1685026146,0,(1,r'''AAAAAADgYEA='''
)),(1229874028,1685026146,0,(1,r'''AAAAAACARkA=''')),(1114793832,1685026146,0,(1,r'''AAAAAAAAWUA='''
)),(1399353968,1685026146,0,(1,r'''AAAAAAAAOUA=''')),(1131180576,1413830740,0,(1,r'''UkdCOiAxMDAuMCUgMTAwLjAlIDEwMC4wJSAoMjU1IDI1NSAyNTUpAA=='''
)),(1935963971,1413830740,0,(1,r'''UkdCOiAwLjAlIDAuMCUgMC4wJSAoMCAwIDApAA==''')),(1111848556
,1413830740,0,(1,r'''QmV2ZWw6IDMvMC4wMDAgMS4wMDAgMC8wLjUwMCAwLjY2MCAwLzEuMDAwIDAuMDAwIDAA'''
))]
})
else:
result = App.Do(Environment, 'MsgBox', {
'Buttons': App.Constants.MsgButtons.OK,
'Icon': App.Constants.MsgIcons.Info,
'Text': 'You need a version of Eye Candy.',
})
Notice that the if statements are placed (left indented) inside the previous if statements, like so:
Code
|----Code
|----Code
|----Code
This is known as nesting. The indentation tells Python when various pieces of code end.